No Content Found
Php Database Column Add Remove Json Object
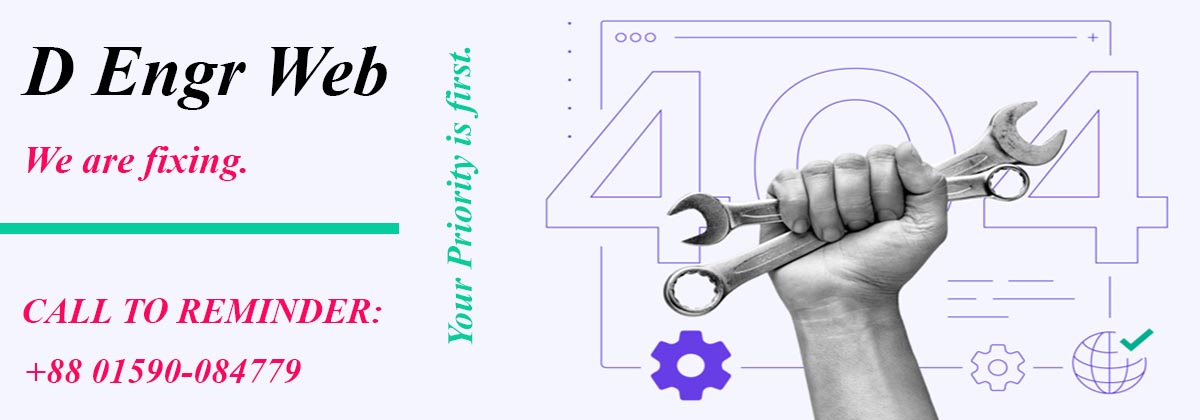
Database column add remove and update by php array object.
Add new items
public function officeNote(Request $request,$orderId)
{
// Validate the incoming request
$request->validate([
'office_note' => 'required|string|max:255', // Adjust validation rules as needed
]);
// Retrieve the order
$order = Order::findOrFail($orderId);
$object_data = $order->office_note ? json_decode($order->office_note) : [];
if (!is_array($object_data)) {
$object_data = [];
}
// Create a new note object
$obj = new \stdClass;
$obj->note = $request->input('office_note');
$obj->user = auth()->user()->name;
$obj->date = Carbon::now()->format('l, F, j Y g:i A');
// Append the new note object to the existing data
$object_data[] = $obj;
// Update and save the order
$order->office_note = json_encode($object_data);
$order->save();
return redirect()->back()->with('success', 'Office note updated successfully.');
}
Remove items by array key
public function officenote_remove($orderId, $key){
$order = Order::findOrFail($orderId);
$object_data = $order->office_note ? json_decode($order->office_note) : [];
if (isset($object_data[$key])) {
unset($object_data[$key]);
}
// Re-index the array to avoid gaps in numeric indices
$object_data = array_values($object_data);
// Encode the updated array back to JSON
$order->office_note = json_encode($object_data);
$order->save();
return redirect()->back()->with('success', 'Office note removed successfully.');
}
```

Write a Associte Comment Markdown Editor
View More Topics

Recent Jobs

View More Categories
